The object models the interest rate curve. More...
#include <interpolatedCurve.hpp>
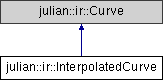
Public Member Functions | |
CurveSettings | getSettings () const |
Returns settings of curve. More... | |
double | getFxSpot () const |
get FxSpot More... | |
double | calculateYF (Date, Date) const |
calculate year fraction More... | |
virtual InterpolatedCurve * | clone () const override |
Virtual copy constructor. More... | |
Constructors | |
InterpolatedCurve () | |
Default constructor. More... | |
InterpolatedCurve (Date today, std::vector< Date > dates, std::vector< double > dfs, InterestRate rate_, SmartPointer< Interpolator > interpolator, SmartPointer< Extrapolator > extrapolator, Calendar calendar, double fx_spot) | |
Constructor. More... | |
InterpolatedCurve (CurveSettings settings, std::vector< Date > dates, std::vector< double > df) | |
Constructor. More... | |
Output getters | |
double | DF (Date) const override |
get DF More... | |
double | DF (Date, Date) const override |
get forward DF More... | |
double | DF (Tenor) const override |
get DF More... | |
double | DF (Tenor, Tenor) const override |
get forward DF More... | |
double | capitalization (Date) const override |
get capitalization More... | |
double | capitalization (Tenor) const override |
get capitalization More... | |
double | capitalization (Date, Date) const override |
get forward capitalization More... | |
double | capitalization (Tenor, Tenor) const override |
get forward capitalization More... | |
double | coupon (Date) const override |
get coupon More... | |
double | coupon (Tenor) const override |
get coupon More... | |
double | coupon (Date, Date) const override |
get coupon More... | |
double | coupon (Tenor, Tenor) const override |
get coupon More... | |
double | rate (Date) const override |
get zero coupon rate More... | |
double | rate (Tenor) const override |
get zero coupon rate More... | |
double | rate (Date, InterestRate) const override |
get zero coupon rate More... | |
double | rate (Tenor, InterestRate) const override |
get zero coupon rate More... | |
double | fwdRate (Date, Date) const override |
get forward rate More... | |
double | fwdRate (Tenor, Tenor) const override |
get forward rate More... | |
double | fwdRate (Date, Tenor) const override |
get forward rate More... | |
double | fwdRate (Date, Date, InterestRate) const override |
get forward rate More... | |
double | fwdRate (Tenor, Tenor, InterestRate) const override |
get forward rate More... | |
double | fwdRate (Date, Tenor, InterestRate) const override |
get forward rate More... | |
std::vector< double > | getDFs () const |
get discount factors More... | |
std::vector< double > | getDFs (std::vector< Date >) const |
get discount factors for given dates More... | |
std::vector< double > | getRates () const |
get zero coupon rates More... | |
std::vector< double > | getRates (InterestRate) const |
get zero coupon rates More... | |
std::vector< double > | getFwdRates () const |
get forward rates More... | |
std::vector< double > | getFwdRates (InterestRate) const |
get forward rates More... | |
Calendar | getCalendar () const |
get calendar More... | |
SmartPointer< Interpolator > | getInterpolator () const |
get interpolator More... | |
SmartPointer< Extrapolator > | getExtrapolator () const |
get extrapolator More... | |
InterestRate | getInterestRate () const |
get interest rate convention More... | |
Getters | |
std::vector< Date > | getDates () const |
get dates vector More... | |
Date | getValuationDate () const override |
get today dates More... | |
Date | getSpotDate () const |
get spot date More... | |
int | getSize () const |
returns the number of nodal dates. More... | |
Setters | |
void | setDates (std::vector< Date >) |
change nodal dates of the curve More... | |
void | setDates (Tenor) |
change nodal dates of the curve More... | |
void | setDFs (std::vector< double >) |
set discount factors More... | |
void | setRates (std::vector< double >) |
set zero coupon rates More... | |
void | setFwdRates (std::vector< double >) |
set forward rate More... | |
void | setFxSpot (double) |
set fx spot for the curve More... | |
![]() | |
Curve () | |
Default constructor. More... | |
virtual | ~Curve () |
destructor More... | |
Private Member Functions | |
template<class Archive > | |
void | serialize (Archive &ar, const unsigned int) |
interface used by Boost serialization library More... | |
Private Attributes | |
Date | today_ |
Today represent the date on which the curve is valid. More... | |
std::vector< Date > | dates_ |
This vector represents grid dates of the curve. More... | |
std::vector< double > | dfs_ |
InterpolatedCurve is represented by values of discount factors for each date. More... | |
InterestRate | rate_ |
The rate encapsulates the concept interest rate (year fraction and compounding). More... | |
SmartPointer< Interpolator > | interpolator_ |
The interpolator encapsulates the concept of interpolation - getting the DF for dates that are not nodes of the time grid. More... | |
SmartPointer< Extrapolator > | extrapolator_ |
The extrapolator encapsulates the concept of extrapolating the curve outside the interval defined by last date of time grid. More... | |
Calendar | calendar_ |
Thanks to calendar outputs of the curve (DFs, ZCRs, etc) can be calculated only on the basis of provided tenor. More... | |
double | fx_spot_ |
Fx Spots allows to calculate two-currency instruments (Fx Forwards and CIRS). More... | |
Friends | |
class | boost::serialization::access |
std::ostream & | operator<< (std::ostream &, InterpolatedCurve &) |
Overloads stream operator. More... | |
Detailed Description
The object models the interest rate curve.
A interest rate curve is a representation of the relationship of rates in the swap or bond markets and their maturity and is used for the valuations of bonds or swap-related instruments. It is usually constructed with benchmark instruments and certain related contracts: market instruments that represent the most liquid and dominant instruments for their respective time horizons. More information see [6] [10] [16] [20] and [41].
The InterpolatedCurve is defined by:
- set of pairs (dates, discount factor) - these represents the concept of time value of money.
- interpolation method - enables to calculate DF for any moment, not just those explicitly given in date grid (see InterestRateCurveInterpolator ).
Additionally, the curve class contains InterestRate object encapsulating the year fraction and compounding convention. The curve is also equipped with calendar and FX rate. Calendar enables to calculate DF, ZCR and Forward Rate on the given tenor. Fx Rate helps in calculating NPV of fx instruments (like FxForward).
It is recommended to build curve by interest rate curve builder ( BuildInterestRateCurve ). This class implements the builder design pattern and enables to build curve on the basis of adequate instruments or directly given discount factors/ zero coupon rates/ forward rates.
Constructor & Destructor Documentation
julian::ir::InterpolatedCurve::InterpolatedCurve | ( | ) |
Default constructor.
julian::ir::InterpolatedCurve::InterpolatedCurve | ( | Date | today, |
std::vector< Date > | dates, | ||
std::vector< double > | dfs, | ||
InterestRate | rate, | ||
SmartPointer< Interpolator > | interpolator, | ||
SmartPointer< Extrapolator > | extrapolator, | ||
Calendar | calendar, | ||
double | fx_spot | ||
) |
Constructor.
julian::ir::InterpolatedCurve::InterpolatedCurve | ( | CurveSettings | settings, |
std::vector< Date > | dates, | ||
std::vector< double > | DFs | ||
) |
Constructor.
Constructor creates curve basing on the CurveSettings provided
Member Function Documentation
calculate year fraction
By this method one can calculate year fraction between to dates. Convention of year fraction is compliant with convention of year fraction of swap curve interest rate.
|
overridevirtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date d.
Implements julian::ir::Curve.
|
overridevirtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date calculated on the basis of given tenor.
Implements julian::ir::Curve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 and received on date d2.
Implements julian::ir::Curve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 (calculated on the basis of tenor t1) and received on date d2 (calculated on the basis of tenor t2).
Implements julian::ir::Curve.
|
overridevirtual |
Virtual copy constructor.
Reimplemented from julian::ir::Curve.
|
overridevirtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d.
Implements julian::ir::Curve.
|
overridevirtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d calculated on basis of tenor t.
Implements julian::ir::Curve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 and received on date d2.
Implements julian::ir::Curve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 calculated on the basis teno1 and received on date d2 calculated on the basis of tenor t2.
Implements julian::ir::Curve.
|
overridevirtual |
get forward DF
This method calculate forward discount factor for a period defined by two dates.
Implements julian::ir::Curve.
|
overridevirtual |
get forward DF
This method calculate discount factor for a period defined by two tenors.
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor t) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
overridevirtual |
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2 (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
|
overridevirtual |
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
|
overridevirtual |
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
Calendar julian::ir::InterpolatedCurve::getCalendar | ( | ) | const |
get calendar
Method returns the calendar defined for the curve.
std::vector< Date > julian::ir::InterpolatedCurve::getDates | ( | ) | const |
get dates vector
The method returns the node points of the curve.
std::vector< double > julian::ir::InterpolatedCurve::getDFs | ( | ) | const |
get discount factors
This method returns discount factors that defines swap curve
std::vector< double > julian::ir::InterpolatedCurve::getDFs | ( | std::vector< Date > | dates | ) | const |
get discount factors for given dates
This method calculates discount factor for dates provided as STL vector.
SmartPointer< ir::Extrapolator > julian::ir::InterpolatedCurve::getExtrapolator | ( | ) | const |
get extrapolator
Method returns the extrapolator defined for the curve.
std::vector< double > julian::ir::InterpolatedCurve::getFwdRates | ( | ) | const |
get forward rates
Method returns forward rates. The first forward rate is the rate between today and first date node. The rest are calculated as rates between nodal points of swap curve.
std::vector< double > julian::ir::InterpolatedCurve::getFwdRates | ( | InterestRate | r | ) | const |
get forward rates
Method returns forward rates. The first forward rate is the rate between today and first date node. The rest are calculated as rates between nodal points of swap curve. The convention of the rates is given as argument of the method.
double julian::ir::InterpolatedCurve::getFxSpot | ( | ) | const |
get FxSpot
Method returns the value of FxSpot.
InterestRate julian::ir::InterpolatedCurve::getInterestRate | ( | ) | const |
get interest rate convention
Method returns the convention of interest rate.
SmartPointer< ir::Interpolator > julian::ir::InterpolatedCurve::getInterpolator | ( | ) | const |
get interpolator
Method returns the interpolator defined for the curve.
std::vector< double > julian::ir::InterpolatedCurve::getRates | ( | ) | const |
get zero coupon rates
This method returns vector of zero coupon rates calculated for grid dates of the curve.
std::vector< double > julian::ir::InterpolatedCurve::getRates | ( | InterestRate | r | ) | const |
get zero coupon rates
This method returns vector of zero coupon rates calculated for grid dates of the curve. The convention of the rates is given as argument of the method.
CurveSettings julian::ir::InterpolatedCurve::getSettings | ( | ) | const |
Returns settings of curve.
Settings are passed using julian::CurveSettings structure
int julian::ir::InterpolatedCurve::getSize | ( | ) | const |
returns the number of nodal dates.
The size of the curve is defined as the number of date nodal points.
Date julian::ir::InterpolatedCurve::getSpotDate | ( | ) | const |
get spot date
The method returns the spot date of the curve.
|
overridevirtual |
get today dates
The method returns the date for which curve was defined.
Implements julian::ir::Curve.
|
overridevirtual |
get zero coupon rate
The zero coupon rate for date d is the yield of zero coupon bond which matures on date d. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
overridevirtual |
get zero coupon rate
The zero coupon rate for tenor t is the yield of zero coupon bond which matures at t. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
overridevirtual |
get zero coupon rate
The zero coupon rate for date d is the yield of zero coupon bond which matures on date d. The rate returned by this method is given in provided interest rate convention.
Implements julian::ir::Curve.
|
overridevirtual |
get zero coupon rate
The zero coupon rate for tenor t is the yield of zero coupon bond which matures at t. The rate returned by this method is given in provided interest rate convention.
Implements julian::ir::Curve.
|
private |
interface used by Boost serialization library
void julian::ir::InterpolatedCurve::setDates | ( | std::vector< Date > | d | ) |
change nodal dates of the curve
Method gives the STL vector of dates. The DFs for this dates are calculated. Then curve is redefined by changing original date grid by new one and changing the DF vector.
void julian::ir::InterpolatedCurve::setDates | ( | Tenor | t | ) |
change nodal dates of the curve
Method provides the tenor. On the basis of this tenor grid date is establish and then this new grid date is used to redefine curve. This method enables to create curve that has fixed inter-space between nodal dates.
void julian::ir::InterpolatedCurve::setDFs | ( | std::vector< double > | input | ) |
set discount factors
This method change DF of the curve.
void julian::ir::InterpolatedCurve::setFwdRates | ( | std::vector< double > | input | ) |
set forward rate
This method change the discount factor of the curve on the basis of forward rates provided.
void julian::ir::InterpolatedCurve::setFxSpot | ( | double | input | ) |
set fx spot for the curve
Sets fx spot rate for a given curve
void julian::ir::InterpolatedCurve::setRates | ( | std::vector< double > | input | ) |
set zero coupon rates
This method change the discount factor of the curve on the basis of zero coupon rates provided.
Friends And Related Function Documentation
|
friend |
Overloads stream operator.
This overloaded operator enables to print the curve on the console.
Member Data Documentation
|
private |
Thanks to calendar outputs of the curve (DFs, ZCRs, etc) can be calculated only on the basis of provided tenor.
|
private |
This vector represents grid dates of the curve.
|
private |
InterpolatedCurve is represented by values of discount factors for each date.
|
private |
The extrapolator encapsulates the concept of extrapolating the curve outside the interval defined by last date of time grid.
|
private |
Fx Spots allows to calculate two-currency instruments (Fx Forwards and CIRS).
|
private |
The interpolator encapsulates the concept of interpolation - getting the DF for dates that are not nodes of the time grid.
|
private |
The rate encapsulates the concept interest rate (year fraction and compounding).
|
private |
Today represent the date on which the curve is valid.
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/interpolatedCurve.hpp
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/interpolatedCurve.cpp