The class interfaces interest rate curves. More...
#include <irCurve.hpp>
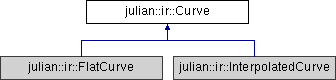
Public Member Functions | |
Curve () | |
Default constructor. More... | |
virtual double | DF (Date) const =0 |
get DF More... | |
virtual double | DF (Date, Date) const =0 |
get forward DF More... | |
virtual double | DF (Tenor) const =0 |
get DF for a tenor More... | |
virtual double | DF (Tenor, Tenor) const =0 |
get forward DF (between two tenors) More... | |
virtual double | capitalization (Date) const =0 |
get capitalization More... | |
virtual double | capitalization (Tenor) const =0 |
get capitalization More... | |
virtual double | capitalization (Date, Date) const =0 |
get forward capitalization More... | |
virtual double | capitalization (Tenor, Tenor) const =0 |
get forward capitalization More... | |
virtual double | coupon (Date) const =0 |
get coupon More... | |
virtual double | coupon (Tenor) const =0 |
get coupon More... | |
virtual double | coupon (Date, Date) const =0 |
get coupon More... | |
virtual double | coupon (Tenor, Tenor) const =0 |
get coupon More... | |
virtual double | rate (Date) const =0 |
get zero coupon rate More... | |
virtual double | rate (Tenor) const =0 |
get zero coupon rate More... | |
virtual double | rate (Date, InterestRate) const =0 |
get zero coupon rate More... | |
virtual double | rate (Tenor, InterestRate) const =0 |
get zero coupon rate More... | |
virtual double | fwdRate (Date, Date) const =0 |
get forward rate More... | |
virtual double | fwdRate (Tenor, Tenor) const =0 |
get forward rate More... | |
virtual double | fwdRate (Date, Tenor) const =0 |
get forward rate More... | |
virtual double | fwdRate (Date, Date, InterestRate) const =0 |
get forward rate More... | |
virtual double | fwdRate (Tenor, Tenor, InterestRate) const =0 |
get forward rate More... | |
virtual double | fwdRate (Date, Tenor, InterestRate) const =0 |
get forward rate More... | |
virtual Date | getValuationDate () const =0 |
get today date More... | |
virtual | ~Curve () |
destructor More... | |
virtual Curve * | clone () const |
Virtual copy constructor. More... | |
Private Member Functions | |
template<class Archive > | |
void | serialize (Archive &ar, const unsigned int) |
interface used by Boost serialization library More... | |
Friends | |
class | boost::serialization::access |
Detailed Description
The class interfaces interest rate curves.
A interest rate curve is a representation of the relationship of rates in the swap or bond markets and their maturity and is used for the valuations of bonds or swap-related instruments. It is usually constructed with benchmark instruments and certain related contracts: market instruments that represent the most liquid and dominant instruments for their respective time horizons. More information see [16] [41] [20] [10] [6] .
Constructor & Destructor Documentation
|
inline |
Default constructor.
|
inlinevirtual |
destructor
Member Function Documentation
|
pure virtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date calculated on the basis of given tenor.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date calculated on the basis of given tenor.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 and received on date d2.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 (calculated on the basis of tenor t1) and received on date d2 (calculated on the basis of tenor t2).
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
virtual |
Virtual copy constructor.
Reimplemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d calculated on basis of tenor t.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 and received on date d2.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 calculated on the basis teno1 and received on date d2 calculated on the basis of tenor t2.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get DF
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward DF
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get DF for a tenor
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward DF (between two tenors)
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor t) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2 (convention of this interest rate is provided as argument of method).
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get today date
The method returns the date for which curve was defined.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get zero coupon rate
The zero coupon rate for date is the yield of zero coupon bond which matures on date. The rate returned by this method is given in curve's interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get zero coupon rate
The zero coupon rate for tenor is the yield of zero coupon bond which matures on given tenor. The rate returned by this method is given in curve's interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get zero coupon rate
The zero coupon rate for date is the yield of zero coupon bond which matures on date d. The rate returned by this method is given in provided interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
pure virtual |
get zero coupon rate
The zero coupon rate for tenor t is the yield of zero coupon bond which matures at t. The rate returned by this method is given in provided interest rate convention.
Implemented in julian::ir::InterpolatedCurve, and julian::ir::FlatCurve.
|
inlineprivate |
interface used by Boost serialization library
The documentation for this class was generated from the following file:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/irCurve.hpp