Implements the second order derivative term of cost function. More...
#include <SecondDerivativeCostFunction.hpp>
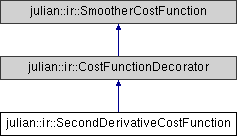
Public Member Functions | |
SecondDerivativeCostFunction (SmartPointer< SmootherCostFunction > c, double weight=1.0) | |
Constructor. More... | |
virtual arma::mat | giveQmatrix (const InterpolatedCurve &) const |
returns Q matrix, the quadratic term of optimization cost function More... | |
virtual arma::mat | giveCvector (const InterpolatedCurve &) const |
returns C vector More... | |
virtual double | calculateCost (const InterpolatedCurve &) const |
returns curve as vector of doubles More... | |
virtual SecondDerivativeCostFunction * | clone () const |
virtual copy constructor More... | |
![]() | |
CostFunctionDecorator (SmartPointer< SmootherCostFunction > c) | |
Constructor. More... | |
virtual std::vector< double > | giveSmoothedCurve (const InterpolatedCurve &) const |
returns curve as vector of doubles More... | |
virtual void | updateSmoothedCurve (InterpolatedCurve &, const std::vector< double > &) const |
updates the curve as with the vector of doubles More... | |
virtual | ~CostFunctionDecorator () |
destructor More... | |
![]() | |
SmootherCostFunction () | |
constructor More... | |
virtual | ~SmootherCostFunction () |
destructor More... | |
Private Attributes | |
double | weight_ |
Weight assigned to second order term (see parameter b in equation above) More... | |
Additional Inherited Members | |
![]() | |
arma::mat | matrixD (int size, int order) const |
Creates matrix representation of differential operator. More... | |
arma::mat | matrixX (const InterpolatedCurve &c, int order) const |
Creates matrix representation of differential operator. More... | |
Detailed Description
Implements the second order derivative term of cost function.
The integral is evaluated numerically:
where is a matrix representing second order differentiation and
are grid dates of the curve.
Constructor & Destructor Documentation
|
inlineexplicit |
Constructor.
Member Function Documentation
|
virtual |
returns curve as vector of doubles
InterpolatedCurve may be represented by different
Reimplemented from julian::ir::CostFunctionDecorator.
|
virtual |
virtual copy constructor
Reimplemented from julian::ir::CostFunctionDecorator.
|
virtual |
returns C vector
Method does not modify the C vector obtained from decorated class.
Reimplemented from julian::ir::CostFunctionDecorator.
|
virtual |
returns Q matrix, the quadratic term of optimization cost function
Numerical derivative of a function f can be approximated using following formula
If then differencing of function can be presented in matrix form:
where:
To calculate Q matrix InterpolatedCurve is needed,because we need the gird dates and the size of the curve
- Parameters
-
input InterpolatedCurve for which Q matrix is calculated
Reimplemented from julian::ir::CostFunctionDecorator.
Member Data Documentation
|
private |
Weight assigned to second order term (see parameter b in equation above)
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/costFunctions/SecondDerivativeCostFunction.hpp
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/costFunctions/SecondDerivativeCostFunction.cpp