Interface for classes decorating SmootherCostFunction. More...
#include <CostFunctionDecorator.hpp>
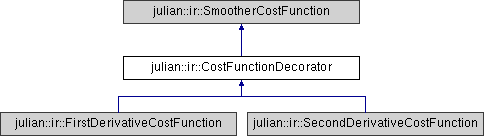
Public Member Functions | |
CostFunctionDecorator (SmartPointer< SmootherCostFunction > c) | |
Constructor. More... | |
virtual arma::mat | giveQmatrix (const InterpolatedCurve &) const |
returns Q matrix, the quadratic term of optimization cost function More... | |
virtual arma::mat | giveCvector (const InterpolatedCurve &) const |
returns C vector, the linear term of optimization cost function More... | |
virtual double | calculateCost (const InterpolatedCurve &) const |
calculates value of cost function More... | |
virtual std::vector< double > | giveSmoothedCurve (const InterpolatedCurve &) const |
returns curve as vector of doubles More... | |
virtual void | updateSmoothedCurve (InterpolatedCurve &, const std::vector< double > &) const |
updates the curve as with the vector of doubles More... | |
virtual CostFunctionDecorator * | clone () const |
virtual copy constructor More... | |
virtual | ~CostFunctionDecorator () |
destructor More... | |
![]() | |
SmootherCostFunction () | |
constructor More... | |
virtual | ~SmootherCostFunction () |
destructor More... | |
Protected Member Functions | |
arma::mat | matrixD (int size, int order) const |
Creates matrix representation of differential operator. More... | |
arma::mat | matrixX (const InterpolatedCurve &c, int order) const |
Creates matrix representation of differential operator. More... | |
Private Attributes | |
SmartPointer< SmootherCostFunction > | cost_function_ |
Pointer to decorator interface. More... | |
Detailed Description
Interface for classes decorating SmootherCostFunction.
CostFunctionDecorator is a interface for all classes decorating the SmootherCostFunction. CostFunctionSmoother's derived classes are used to define the cost function of optimization problems used in ir::InterpolatedCurve construction.
Class also contains methods to calculate the D matrix and X matrix that implements differential operator.
Constructor & Destructor Documentation
|
inlineexplicit |
Constructor.
|
inlinevirtual |
destructor
Member Function Documentation
|
virtual |
calculates value of cost function
Implements julian::ir::SmootherCostFunction.
Reimplemented in julian::ir::FirstDerivativeCostFunction, and julian::ir::SecondDerivativeCostFunction.
|
virtual |
virtual copy constructor
Implements julian::ir::SmootherCostFunction.
Reimplemented in julian::ir::FirstDerivativeCostFunction, and julian::ir::SecondDerivativeCostFunction.
|
virtual |
returns C vector, the linear term of optimization cost function
Implements julian::ir::SmootherCostFunction.
Reimplemented in julian::ir::FirstDerivativeCostFunction, and julian::ir::SecondDerivativeCostFunction.
|
virtual |
returns Q matrix, the quadratic term of optimization cost function
Implements julian::ir::SmootherCostFunction.
Reimplemented in julian::ir::FirstDerivativeCostFunction, and julian::ir::SecondDerivativeCostFunction.
|
virtual |
returns curve as vector of doubles
InterpolatedCurve may be represented by different
Implements julian::ir::SmootherCostFunction.
|
protected |
Creates matrix representation of differential operator.
Numerical derivative of a function f can be approximated using following formula
If then differencing of function can be presented in matrix form"
where:
The second derivative can be calculated by analogy.
This method calculates the D matrix.
- Parameters
-
size Size of D matrix derivative Order of derivative
|
protected |
Creates matrix representation of differential operator.
Numerical derivative of a function f can be approximated using following formula
If then differencing of function can be presented in matrix form:
where:
The second derivative can be calculated by analogy.
This method calculates the X matrix.
- Parameters
-
c Curve which derivative we calculates order Order of derivative
|
virtual |
updates the curve as with the vector of doubles
Implements julian::ir::SmootherCostFunction.
Member Data Documentation
|
private |
Pointer to decorator interface.
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/costFunctions/CostFunctionDecorator.hpp
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/costFunctions/CostFunctionDecorator.cpp