Interest rate curve estimating algorithm using the derivative root finder. More...
#include <rootFindingBootstrapper.hpp>
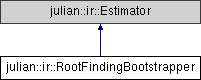
Public Member Functions | |
RootFindingBootstrapper (DerivativeRootFinder method=DerivativeRootFinder::STEFFENSEN) | |
constructor More... | |
void | calculate (const std::vector< SmartPointer< BuildingBlock > > &instruments, const CurveSettings &settings, SmartPointer< ir::Curve > &discounting, SmartPointer< ir::Curve > &projection) |
estimates the curve More... | |
std::vector< double > | getDF () const |
returns DFs More... | |
std::vector< Date > | getDates () const |
returns dates More... | |
std::string | info () const |
returns name of estimator More... | |
RootFindingBootstrapper * | clone () const |
virtual copy constructor More... | |
![]() | |
Estimator () | |
default constructor More... | |
virtual | ~Estimator () |
destructor More... | |
Private Attributes | |
std::vector< double > | discount_factors_ |
Vector of discount factors. More... | |
std::vector< Date > | dates_ |
Vector of grid dates. More... | |
DerivativeRootFinder | method_ |
Algorithm type. More... | |
Detailed Description
Interest rate curve estimating algorithm using the derivative root finder.
Class implements the algorithm estimating interest rate curves using the derivative root finder (compare DerivativeRootFinder). The curve is estimated as follows:
1) Setting first grid point: today dates and DF1 = 1. Reading the first (with shortest maturity) instrument ( ) and getting its maturity date
.
2) Creating new curve with dates
and discount factors
3) Solving function f1 using root finding algorithm: .
4) Reading the next instrument ( ) with maturity
. Creating new curve
with dates
and discount factors
5) Solving function f2 using root finding algorithm: .
6) Reading the next instrument ( ) with maturity
. Creating new curve
with dates
and discount factors
7) Solving function f3 using root finding algorithm: .
etc.
The derivative of f function is calculated numerically using finite difference approximation. If discounting curve and projection curve are provided, they are used when instrument is priced. This approach enables estimating the curve in the multi-curve framework (see [26]).
- Warning
- Estimator does not work properly with FlatBackward interpolation. RootFindingBootstrapper estimates curve by varying last DF. In FlatBackward the shape of the curve does not depend on last DF so calibration cannot be performed
- Examples:
- bootstrapperComparison.cpp, and rootFindingBootstrapperExample.cpp.
Constructor & Destructor Documentation
|
inline |
constructor
Member Function Documentation
|
virtual |
estimates the curve
calculates method estimates the curve and saves the result into the class members
Implements julian::ir::Estimator.
|
virtual |
virtual copy constructor
Implements julian::ir::Estimator.
|
virtual |
returns dates
Method should be called after calling method Estimator::calculate
Implements julian::ir::Estimator.
|
virtual |
returns DFs
Method should be called after calling method Estimator::calculate
Implements julian::ir::Estimator.
|
virtual |
returns name of estimator
Implements julian::ir::Estimator.
Member Data Documentation
|
private |
Vector of grid dates.
|
private |
Vector of discount factors.
|
private |
Algorithm type.
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/rootFindingBootstrapper.hpp
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/estimators/rootFindingBootstrapper.cpp