The class defines the flat interest rate curve. More...
#include <flatCurve.hpp>
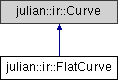
Public Member Functions | |
FlatCurve () | |
default constructor More... | |
FlatCurve (InterestRate &, double, Date, Calendar &) | |
constructor More... | |
virtual double | DF (Date) const |
get DF More... | |
virtual double | DF (Date, Date) const |
get forward DF More... | |
virtual double | DF (Tenor) const |
get DF for a tenor More... | |
virtual double | DF (Tenor, Tenor) const |
get forward DF (between two tenors) More... | |
virtual double | capitalization (Date) const |
get capitalization More... | |
virtual double | capitalization (Tenor) const |
get capitalization More... | |
virtual double | capitalization (Date, Date) const |
get forward capitalization More... | |
virtual double | capitalization (Tenor, Tenor) const |
get forward capitalization More... | |
virtual double | coupon (Date) const |
get coupon More... | |
virtual double | coupon (Tenor) const |
get coupon More... | |
virtual double | coupon (Date, Date) const |
get coupon More... | |
virtual double | coupon (Tenor, Tenor) const |
get coupon More... | |
virtual double | rate (Date) const |
get zero coupon rate More... | |
virtual double | rate (Tenor) const |
get zero coupon rate More... | |
virtual double | rate (Date, InterestRate) const |
get zero coupon rate More... | |
virtual double | rate (Tenor, InterestRate) const |
get zero coupon rate More... | |
virtual double | fwdRate (Date, Date) const |
get forward rate More... | |
virtual double | fwdRate (Tenor, Tenor) const |
get forward rate More... | |
virtual double | fwdRate (Date, Tenor) const |
get forward rate More... | |
virtual double | fwdRate (Date, Date, InterestRate) const |
get forward rate More... | |
virtual double | fwdRate (Tenor, Tenor, InterestRate) const |
get forward rate More... | |
virtual double | fwdRate (Date, Tenor, InterestRate) const |
get forward rate More... | |
virtual Date | getValuationDate () const |
get today date More... | |
FlatCurve * | clone () const |
Virtual copy constructor. More... | |
![]() | |
Curve () | |
Default constructor. More... | |
virtual | ~Curve () |
destructor More... | |
Private Member Functions | |
template<class Archive > | |
void | serialize (Archive &ar, const unsigned int) |
interface used by Boost serialization library More... | |
Private Attributes | |
InterestRate | rate_ |
Interest rate convention. More... | |
double | zero_coupon_rate_ |
Value of zero coupon rate. More... | |
Date | curve_date_ |
Today represent the date on which the curve is valid. More... | |
Calendar | calendar_ |
Thanks to calendar outputs of the curve (DFs, forward rates etc) can be calculated only on the basis of provided tenor. More... | |
Friends | |
class | boost::serialization::access |
std::ostream & | operator<< (std::ostream &, FlatCurve &) |
Overloads stream operator. More... | |
Detailed Description
The class defines the flat interest rate curve.
Flat interest rate curve is a curve which Zero Coupon rate is the same for all tenors.
- Examples:
- bondsExample.cpp, DepositExample.cpp, and optionPricingExample.cpp.
Constructor & Destructor Documentation
julian::ir::FlatCurve::FlatCurve | ( | ) |
default constructor
julian::ir::FlatCurve::FlatCurve | ( | InterestRate & | rate, |
double | zero_coupon_rate, | ||
Date | curve_date, | ||
Calendar & | calendar | ||
) |
constructor
Member Function Documentation
|
virtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date calculated on the basis of given tenor.
Implements julian::ir::Curve.
|
virtual |
get capitalization
This method calculate the value of 1 monetary unit invested today and received on date calculated on the basis of given tenor.
Implements julian::ir::Curve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 and received on date d2.
Implements julian::ir::Curve.
get forward capitalization
This method calculate the value of 1 monetary unit invested on date d1 (calculated on the basis of tenor t1) and received on date d2 (calculated on the basis of tenor t2).
Implements julian::ir::Curve.
|
virtual |
Virtual copy constructor.
Reimplemented from julian::ir::Curve.
|
virtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d.
Implements julian::ir::Curve.
|
virtual |
get coupon
This method calculate the revenue on 1 monetary unit invested today and received on date d calculated on basis of tenor t.
Implements julian::ir::Curve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 and received on date d2.
Implements julian::ir::Curve.
get coupon
This method calculate the revenue on 1 monetary unit invested on date d1 calculated on the basis teno1 and received on date d2 calculated on the basis of tenor t2.
Implements julian::ir::Curve.
|
virtual |
get DF
Implements julian::ir::Curve.
get forward DF
Implements julian::ir::Curve.
|
virtual |
get DF for a tenor
Implements julian::ir::Curve.
get forward DF (between two tenors)
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor t) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
virtual |
get forward rate
The forward rate between d1 and d2 is the yield of zero coupon bond that will commence at date d1 and matures at date d2 (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
|
virtual |
get forward rate
The forward rate between d1 and d2 ( date calculated on the basis of provided tenors) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
|
virtual |
get forward rate
The forward rate between d1 and d2 ( date d2 calculated on the basis of provided tenor) is the yield of zero coupon bond that will commence at date d1 and matures at date d2. (convention of this interest rate is provided as argument of method).
Implements julian::ir::Curve.
|
virtual |
get today date
The method returns the date for which curve was defined.
Implements julian::ir::Curve.
|
virtual |
get zero coupon rate
The zero coupon rate for date is the yield of zero coupon bond which matures on date. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
virtual |
get zero coupon rate
The zero coupon rate for tenor is the yield of zero coupon bond which matures on given tenor. The rate returned by this method is given in curve's interest rate convention.
Implements julian::ir::Curve.
|
virtual |
get zero coupon rate
The zero coupon rate for date is the yield of zero coupon bond which matures on date d. The rate returned by this method is given in provided interest rate convention.
Implements julian::ir::Curve.
|
virtual |
get zero coupon rate
The zero coupon rate for tenor t is the yield of zero coupon bond which matures at t. The rate returned by this method is given in provided interest rate convention.
Implements julian::ir::Curve.
|
private |
interface used by Boost serialization library
Friends And Related Function Documentation
|
friend |
Overloads stream operator.
This overloaded operator enables to print the curve on the console.
Member Data Documentation
|
private |
Thanks to calendar outputs of the curve (DFs, forward rates etc) can be calculated only on the basis of provided tenor.
|
private |
Today represent the date on which the curve is valid.
|
private |
Interest rate convention.
|
private |
Value of zero coupon rate.
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/flatCurve.hpp
- C:/Unix/home/OEM/jULIAN/src/marketData/interestRateCurves/flatCurve.cpp