Class implements simple linear regression. More...
#include <simpleLinearRegression.hpp>
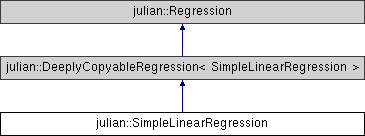
Public Member Functions | |
void | estimate (const std::vector< double > &x, const std::vector< double > &y) |
estimates the parameters basing on provided data More... | |
void | estimate (const std::vector< double > &x, const std::vector< double > &y, const std::vector< double > &w) |
estimates the parameters basing on provided data and weights More... | |
double | getSlope () |
returns slope More... | |
double | getIntercept () |
returns intercept More... | |
std::vector< double > | getCoefficient () const |
return coefficients of the regression More... | |
double | operator() (double) const |
evaluates the linear regression model for x More... | |
![]() | |
virtual Regression * | clone () const |
virtual copy constructor More... | |
![]() | |
Regression () | |
Constructor. More... | |
virtual | ~Regression () |
Destructor. More... | |
Private Attributes | |
double | chi_sq_ |
The sum of squares of the residuals from the best-fit line. More... | |
double | cov00_ |
Variance of the slope estimate. More... | |
double | cov01_ |
Covariance of the slope/intercept estimates. More... | |
double | cov11_ |
Variance of the intercept estimate. More... | |
double | c0_ |
Intercept of linear regression. More... | |
double | c1_ |
Slope of linear regression. More... | |
Detailed Description
Class implements simple linear regression.
Simple linear regression is a linear regression model with a single explanatory variable. The parameters of the regression are calculated by minimizing the OLS. In other words class computes the best-fit linear regression coefficients of the model
for the dataset (x, y) by minimizing the sum:
For more information see Wikipedia
- Remarks
- Class uses algorithm implemented in GSL
Member Function Documentation
|
virtual |
estimates the parameters basing on provided data
Implements julian::Regression.
void julian::SimpleLinearRegression::estimate | ( | const std::vector< double > & | x, |
const std::vector< double > & | y, | ||
const std::vector< double > & | w | ||
) |
estimates the parameters basing on provided data and weights
|
virtual |
return coefficients of the regression
The i-th term of vector represents coefficient of
Implements julian::Regression.
double julian::SimpleLinearRegression::getIntercept | ( | ) |
returns intercept
double julian::SimpleLinearRegression::getSlope | ( | ) |
returns slope
|
virtual |
evaluates the linear regression model for x
Implements julian::Regression.
Member Data Documentation
|
private |
Intercept of linear regression.
|
private |
Slope of linear regression.
|
private |
The sum of squares of the residuals from the best-fit line.
|
private |
Variance of the slope estimate.
|
private |
Covariance of the slope/intercept estimates.
|
private |
Variance of the intercept estimate.
The documentation for this class was generated from the following files:
- C:/Unix/home/OEM/jULIAN/src/mathematics/regressions/simpleLinearRegression.hpp
- C:/Unix/home/OEM/jULIAN/src/mathematics/regressions/simpleLinearRegression.cpp